Introduction
Controlling an appliance using a 5V SPDT relay module and an ESP32-WROOM microcontroller is a very common application used in everyday home automation and consumer electronics, allowing a foundational understanding of DIY home automation projects. In today’s tutorial, we are going to understand how to control any appliance wirelessly using an SPDT relay and an ESP32-WROOM microcontroller.
What is a Relay?
A SPDT relay is an electromechanical switch device that operates using a 5V DC voltage applied to its coil. It has one common terminal (COM) that can be connected to either a normally open (NO) or normally closed (NC) contact, depending on the relay’s state. When the 5V supply energizes the coil, it generates a magnetic field, causing the internal switch contacts to switch between these positions.
Hardware Components
You’ll need the following hardware components to get started:
Components | Value / Model | Qty |
---|---|---|
ESP32 | – | 1 |
Push Button | – | 1 |
Relay | 5v | 1 |
Power Adapter | 12v | 1 |
DC Power Jack | – | 1 |
Breadboard | – | 1 |
Jumper Wires | – | 1 |
DC Power for ESP32 | – | 1 |
Steps-by-Step Guide
(1) Setting up Arduino IDE
Download Arduino IDE Software from its official site. Here is a step-by-step guide on “How to install Arduino IDE“.
(2) ESP32 in Arduino IDE
There’s an add-on that allows you to program the ESP32 using the Arduino IDE. Here is a step-by-step guide on “How to Install ESP32 on Arduino IDE“.
(3) Schematic
Make connections according to the circuit diagram given below.
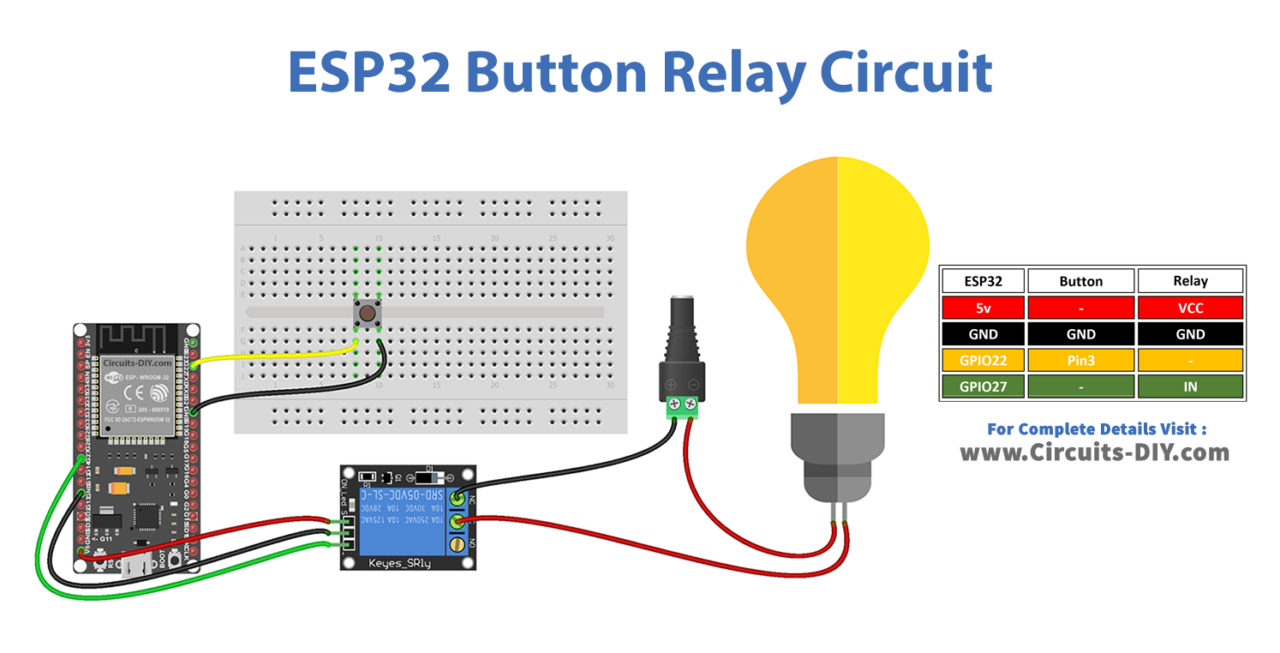
Wiring / Connections
ESP32 | Button | Relay |
---|---|---|
5V | – | VCC |
GND | GND | GND |
GIOP22 | Pin 3 | – |
GIOP27 | – | IN |
(4) Uploading Code
Now copy the following code and upload it to Arduino IDE Software.
#define BUTTON_PIN 22 // ESP32 pin GIOP22 connected to button's pin
#define RELAY_PIN 27 // ESP32 pin GIOP27 connected to relay's pin
void setup() {
Serial.begin(9600); // initialize serial
pinMode(BUTTON_PIN, INPUT_PULLUP); // set ESP32 pin to input pull-up mode
pinMode(RELAY_PIN, OUTPUT); // set ESP32 pin to output mode
}
void loop() {
int buttonState = digitalRead(BUTTON_PIN); // read new state
if (buttonState == LOW) {
Serial.println("The button is being pressed");
digitalWrite(RELAY_PIN, HIGH); // turn on
}
else if (buttonState == HIGH) {
Serial.println("The button is unpressed");
digitalWrite(RELAY_PIN, LOW); // turn off
}
}